DataGridView开发例子一个
DataGridView开发例子
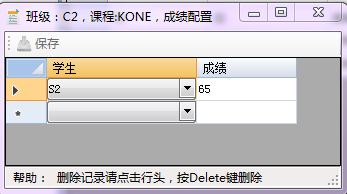
使用BindingSource来绑定数据
功能:学生成绩的录入,
要求
- 一个学生一条记录
Public Class FMCourse
Private _CourseList As DBList(Of Student_Courses)
Private _DA As Student_CoursesDA
Private _StudentsList As List(Of Base_Students)
''' <summary>
''' 窗体关闭时,释放一些对像
''' </summary>
''' <param name="sender"></param>
''' <param name="e"></param>
''' <remarks></remarks>
Private Sub FMCourses_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
If (_DA IsNot Nothing) Then
_DA.Dispose()
End If
_DA = Nothing
_CourseList = Nothing
End Sub
''' <summary>
''' 窗体打开时,初始化对像
''' </summary>
''' <param name="sender"></param>
''' <param name="e"></param>
''' <remarks></remarks>
Private Sub FMCourses_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
xDataGridView.AutoGenerateColumns = False
Me.Text = String.Format("班级:{0},课程:{1},成绩配置", My.Forms.FMMain.mCondition1_Classs.ClassName, My.Forms.FMMain.mCondition1_Lesson.LessonName)
BindSelect()
LoadData()
checkControl()
End Sub
''' <summary>
''' 绑定选择项
''' </summary>
''' <remarks></remarks>
Private Sub BindSelect()
Using pDA As New Base_StudentsDA
BaseStudentsUserGIDDataGridViewColumn.DisplayMember = Base_Students.DisplayMember
BaseStudentsUserGIDDataGridViewColumn.ValueMember = Base_Students.ValueMember
pDA.mCondition.Base_ClassClassGID = My.Forms.FMMain.mCondition1_Classs.ClassGID
Dim pStudentsList As List(Of Base_Students) = pDA.mFunctions.getListByCondition("ClassGID") '导入指定班级的学生
_StudentsList = pStudentsList.ToList()
pStudentsList.Insert(0, Base_Students.CreateBase_Students(Guid.Empty, "未选择", "", "", "", False, "", "", "", Guid.Empty)) '作为默认值显示
BaseStudentsUserGIDDataGridViewColumn.DataSource = pStudentsList
End Using
End Sub
''' <summary>
''' 导入已经添加的数据
''' </summary>
''' <remarks></remarks>
Private Sub LoadData()
Try
_DA = New Student_CoursesDA()
_DA.mCondition.Base_LessonLessonCode = My.Forms.FMMain.mCondition1_Lesson.LessonCode
_DA.mCondition.Base_ClassClassGID = My.Forms.FMMain.mCondition1_Classs.ClassGID
_CourseList = _DA.mFunctions.getDBListByCondition("LessonAndClass")
'在绑定到表格前,要先检查一遍学生列表,如学生已不在该班级,就用默认值代替
For Each pCourse In _CourseList
Dim pCourse2 As Student_Courses = pCourse
If (_StudentsList.Exists(Function(p) p.UserGID = pCourse2.Base_StudentsUserGID) = False) Then
pCourse.Base_StudentsUserGID = Guid.Empty
End If
Next
StudentCoursesBindingSource.DataSource = _CourseList '绑定数据到表格
XDataGridView.Refresh()
Catch ex As Exception
MsgBox(ex.Message)
End Try
End Sub
Private Sub xSave_Click(sender As System.Object, e As System.EventArgs) Handles xSave.Click
XDataGridView.EndEdit()
StudentCoursesBindingSource.EndEdit()
Application.DoEvents()
If (xDataGridView.IsCurrentRowDirty = False) Then '在保存之前,要保证所有数据已符合要求,如果有数据不能符合要求,则不执行提交保存
_DA.submit(_CourseList)
checkControl()
Else
Me.xHelp.Text = "请检查数据正确后,再点击保存"
End If
End Sub
Private Sub StudentCoursesBindingSource_AddingNew(sender As System.Object, e As System.ComponentModel.AddingNewEventArgs) Handles StudentCoursesBindingSource.AddingNew
'添加一条新记录,并设置一些默认值
Dim pClass As Student_Courses = Student_Courses.CreateStudent_Courses(0, Guid.Empty, My.Forms.FMMain.mCondition1_Lesson.LessonCode, 0, My.Forms.FMMain.mCondition1_Classs.ClassGID)
e.NewObject = pClass
checkControl()
End Sub
''' <summary>
''' 用户删除数据时,BindingSource会自动从列表中删除,在提交保存时自动从数据库中删除,此处只要检查删除后的控件状态
''' </summary>
''' <param name="sender"></param>
''' <param name="e"></param>
''' <remarks></remarks>
Private Sub XDataGridView_UserDeletedRow(sender As Object, e As System.Windows.Forms.DataGridViewRowEventArgs) Handles xDataGridView.UserDeletedRow
checkControl()
End Sub
Private Sub XDataGridView_UserDeletingRow(sender As System.Object, e As System.Windows.Forms.DataGridViewRowCancelEventArgs) Handles xDataGridView.UserDeletingRow
If (MsgBox("确认要删除这条记录吗?", vbYesNo, My.Application.Info.Title) = MsgBoxResult.No) Then
e.Cancel = True
Return
End If
End Sub
''' <summary>
''' 检查控件状态以及进行一些帮助提示
''' </summary>
''' <remarks></remarks>
Private Sub checkControl()
xSave.Enabled = False
If (_CourseList IsNot Nothing) Then
For Each pCourse In _CourseList
If (pCourse.Base_StudentsUserGID = Guid.Empty) Then
xHelp.Text = "请选择学生信息"
Return
End If
Next
If (_CourseList.submitRequird) Then
xHelp.Text = "数据已准备完毕,可以保存"
xSave.Enabled = True
End If
End If
End Sub
''' <summary>
''' 对表格数据进行检查,不符合要求,不允许提交更改
''' </summary>
''' <param name="sender"></param>
''' <param name="e"></param>
''' <remarks></remarks>
Private Sub xDataGridView_RowValidating(sender As System.Object, e As System.Windows.Forms.DataGridViewCellCancelEventArgs) Handles xDataGridView.RowValidating
If (_CourseList Is Nothing) Then
Return
End If
If (e.RowIndex < xDataGridView.Rows.Count) Then
Dim pValue As Object = xDataGridView.Rows(e.RowIndex).Cells(BaseStudentsUserGIDDataGridViewColumn.Index).Value
If (pValue IsNot Nothing) Then
'判断同一个学生只能出现一次,不能同一门课出现2个成绩
Dim pCount As Integer = _CourseList.Where(Function(p) p.Base_StudentsUserGID = pValue).Count
If (pCount > 1) Then
Me.xHelp.Text = "同一个学生只能有一个成绩"
Me.xHelp.ForeColor = Color.Red
xDataGridView.EndEdit()
e.Cancel = True
Return
End If
End If
End If
Me.xHelp.Text = "删除记录请点击行头,按Delete键删除"
xHelp.ForeColor = Color.Black
End Sub
''' <summary>
''' 通过检查的数据,设置数据的变动状态,以便于在提交保存时,能把数据修改同步到数据库
''' </summary>
''' <param name="sender"></param>
''' <param name="e"></param>
''' <remarks></remarks>
Private Sub xDataGridView_RowValidated(sender As System.Object, e As System.Windows.Forms.DataGridViewCellEventArgs) Handles xDataGridView.RowValidated
If (_CourseList Is Nothing OrElse xDataGridView.IsCurrentRowDirty = False) Then
Return
End If
'用户编辑完后,设置一下数据状态
Dim pItem As Object = xDataGridView.Rows(e.RowIndex).DataBoundItem
If (pItem IsNot Nothing) Then
Dim pCourses As Student_Courses = CType(pItem, Student_Courses)
pCourses.autoChangeRowState()
End If
checkControl()
End Sub
End Class